When you trade in the global foreign exchange market, you know that some hours are better than others. Some currency pairs are more active during specific time intervals, and sometimes, you even want to avoid trading during particular periods of the day. In this guide, you will see how to use MetaTrader 4 platform and MQL4 language to limit Forex trading (and not only trading) during specific hours.

Forex Trading Hours
As you may already know, Forex is a 24-hour market. The currency market stops only for a few hours during the weekend. The rest of the time it is open and active. With Forex being a global decentralized market, you can always find trading opportunities in any area of the world and at any time of the day. Having said that, you must also know that there are time periods when the market is more active than during the others. For example, the London open at 8 AM London time and the overlap of the European session and New York open after 8 AM New York time are the most active periods for EUR, USD, and GBP trading.
Having a market that is open for 24 hours a day allows many traders to participate, and each trader can find some opportunity. For example:
- Think about a trader living in Sydney with a full-time job and working during business hours. Due to the difference in time zone, that trader can easily participate during the European session focusing on EUR, GPB, and CHF.
- Think about another trader living in Miami, busy during business hour with their day job. This trader can choose to trade the Asian session.
The 24-hour market surely gives many advantages, but what if your trading is automated? That's even better! Your trading strategy is run by a personal computer (or a server) and can be executed 24 hours non-stop. Some strategies though work better only during some hours of the day, and in this case, you need to find the way to enable your activity only during those periods.
How to Limit Forex Trading Only to Specific Time Period?
MetaTrader 4 can run your Forex trading strategy with an expert advisor all day, every day. And with MQL4, you can decide to execute some of the activities only during specific time intervals.
Enabling the execution of tasks during only some hours is not too difficult, it just needs coding some comparisons. As in many other cases, MQL4 provides useful tools to achieve your goal; in this case, the tool is the function Hour()
. The Hour()
function returns the last know hour of the MetaTrader server time. If your platform is connected to the server, this is the current hour, with an integer value from 0 to 23.
Please remember that Hour()
returns the value of the hour on the server, which, in case you are in a different time zone, will be different from your local time.
In MetaTrader, you can see the server time in the Market Watch panel:
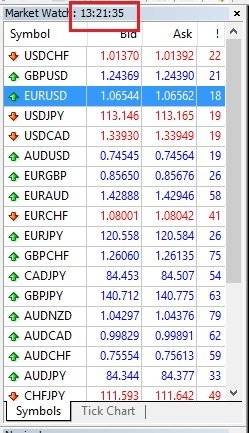
You can set up a script or an expert advisor with two input parameters:
- Start Hour — a number from 0 to 23; it will be the start of trading operations.
- Last Hour — a number from 0 to 23 to set the last hour of operations (inclusively).
If Start Hour and Last Hour are the same, then trading is allowed only during that hour. Start Hour can be before or after Last Hour — in this case, operations will be enabled overnight.
Example: Start Hour = 7, Last Hour = 19. Operations enabled between 7 AM and 7 PM.
Example: Start Hour = 20, Last Hour = 6. Operations enabled from 8 PM to 6 AM.
The code to perform the check is the following:
//+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ #property strict #property show_inputs // Definition of an hour. This is necessary for a drop down menu for hours input. enum ENUM_HOUR { h00 = 00, // 00:00 h01 = 01, // 01:00 h02 = 02, // 02:00 h03 = 03, // 03:00 h04 = 04, // 04:00 h05 = 05, // 05:00 h06 = 06, // 06:00 h07 = 07, // 07:00 h08 = 08, // 08:00 h09 = 09, // 09:00 h10 = 10, // 10:00 h11 = 11, // 11:00 h12 = 12, // 12:00 h13 = 13, // 13:00 h14 = 14, // 14:00 h15 = 15, // 15:00 h16 = 16, // 16:00 h17 = 17, // 17:00 h18 = 18, // 18:00 h19 = 19, // 19:00 h20 = 20, // 20:00 h21 = 21, // 21:00 h22 = 22, // 22:00 h23 = 23, // 23:00 }; input ENUM_HOUR StartHour = h07; // Start operation hour input ENUM_HOUR LastHour = h17; // Last operation hour bool CheckActiveHours() { // Set operations disabled by default. bool OperationsAllowed = false; // Check if the current hour is between the allowed hours of operations. If so, return true. if ((StartHour == LastHour) && (Hour() == StartHour)) OperationsAllowed = true; if ((StartHour < LastHour) && (Hour() >= StartHour) && (Hour() <= LastHour)) OperationsAllowed = true; if ((StartHour > LastHour) && ((Hour() <= LastHour) || (Hour() >= StartHour))) OperationsAllowed = true; return OperationsAllowed; } void OnStart() { if (CheckActiveHours()) Print("Trading enabled"); } //+------------------------------------------------------------------+
As you can see the code is pretty easy, you can check if the current hour is included in the hour of operations and return true
or false
, you can then use that true
or false
to limit the execution of a task.
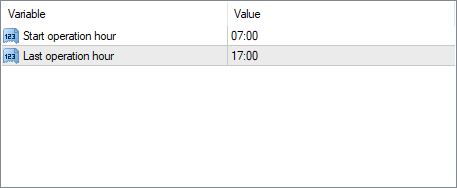
You can use our Trading Session Time indicator for MetaTrader to highlight specific zones on charts. This will help you visualize the periods when you don't want to trade along with the periods when you want trading to be enabled.
Conclusion
Because the Forex market is open 24 hours almost 7 days a week, you may want to restrict your trading operations to only specific time intervals during the day. It is quite easy to limit execution of tasks in an expert advisor to specific times using MetaTrader 4 and MQL4 language. Above you saw a practical example.
If you are interested in the topic of EA optimization, you can also learn about optimizing expert advisors based on days of the week.