In this guide, you will learn how to get the current price using the MQL4 language.
When coding an indicator or an expert advisor, it is often necessary to get the current price via MQL language. MQL4 get current price functions are very simple and in this guide you are going to see some code to achieve this.
Get Current Price with MQL4
Getting the current price of a currency pair or another trading instrument is one of the frequent tasks to perform when coding a new indicator or expert advisor. The operation itself is very easy and we are going to examine the code to do that.
Before coding, it is first necessary to establish what price we need to extract. Each instrument has in fact two prices: Bid and Ask. Bid is the price you can sell an instrument at, while Ask is the price you pay to buy it.
When in MetaTrader 4 we see the current price line on a chart, that is by default the Bid price.
MQL4 Get Current Price Using MarketInfo()
The following code uses the native MetaTrader 4 function MarketInfo()
to get the price of the current trading instrument. The values of Bid and Ask are then saved into variables and presented within a popup text box.
You can test the code by simply creating a new script and copying/pasting it:
void OnStart() { // MQL Get Current Price. // Get Ask and Bid of the current pair with MarketInfo // and save the values in variables. double PriceAsk = MarketInfo(Symbol(), MODE_ASK); double PriceBid = MarketInfo(Symbol(), MODE_BID); // Showing a message box with the values. MessageBox("Bid = " + DoubleToString(PriceBid, Digits) + " Ask = " + DoubleToString(PriceAsk, Digits)); }
And here is the result of the script's execution:
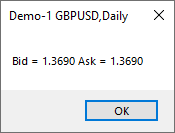
MarketInfo()
is a very powerful function that can return a lot of information about an instrument. In the way we used it, we just asked it to retrieve the Bid and Ask price for the current chart's asset.
MQL4 Get Current Price with Close()
There is an alternative way of retrieving the current price, but in this case, we will only be able to get the current Bid price via MQL4 code. This alternative method is using the function Close()
or iClose()
.
Close()
is a function that returns the Close price (Bid) of the selected candle, where the selection is done via a index. Close[0]
will return the Close price of the candle at index 0, which is the one in formation — the current candle.
Using this function we can come with the following piece of code:
void OnStart() { // MQL Get Current Price. // Get Bid of the current pair with Close() // and save the value in a variable. double PriceBid = Close[0]; // Showing a message box with the value. MessageBox("Bid = " + PriceBid); }
Conclusion
As you can see, it isn't hard to get the current price via MQL4 code. This only requires a few lines of code. The MQL4 language already includes tools to help achieving it, and it is just a matter of finding and using them.